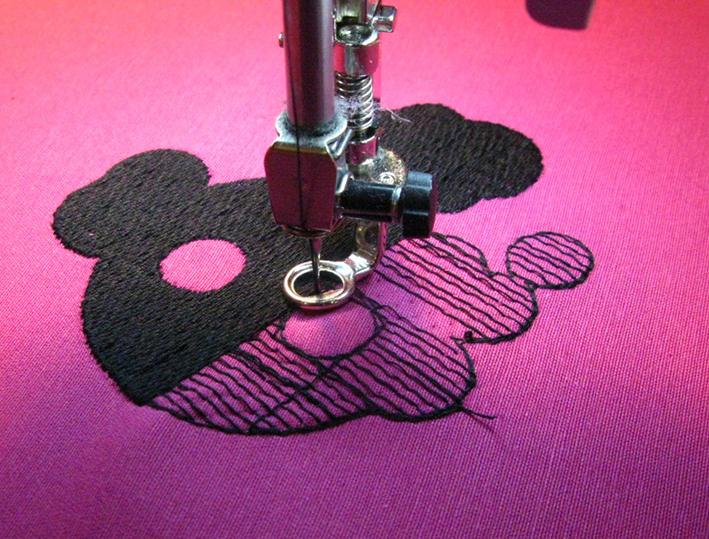
I wanted to write a program that would generate different faces. I have started with the “tree” source code that Leah has provide for the embroidery machine toutorial. I was changing the properties untill I found the result close enough my idea. I picked up three random face-bases and I added the eyes in Adobe Illustrator.
The embroidery machine was quite nice to me, however I needed some help to tame the bobbin. Sheralyn gave me her great support today, (Thank you!). My first version on a white fabric was a faces trial. I wasn’t satisfied with the scale and composition so I adjusted my pattern in Illutrator and tried the second time on the pink fabric.
Most surprinig for mye was the machine’s “embroiding concept”. As I am used to the pronters I expected the same linear movement of the needle. It was interesting to see (and hard to understand) why the machine chose some pieces to draw first even if they were away from each other. I appreciate that we had an opportunity to lear this new technique in combination with programing in Processing.
Processing Code:
//Based on Leah’s Buechley Tree Generator
import processing.pdf.*;
float theta;
void setup() {
//set the size of the window (& the pdf document)
size(640, 600);
smooth();
//set the name of the pdf document
beginRecord(PDF, “tree.pdf”);
}
void draw() {
//everything happens in keyPressed
}
void keyPressed() {
//if the ‘q’ key is pressed, finish drawing the PDF & quit
if (key == ‘q’) {
endRecord();
exit();
}
//if any other key is pressed, draw a new face
else {
//set the background color to be white
background(255);
//set the stroke to be black
stroke(0);
//start the face in the middle of the screen
translate(width/2,height/2);
// Draw a circle
ellipse(0,0,60,60);
// Move to the edge
translate(0,-25);
// Start growing hair!
hair(30);
}
}
void hair(float h) {
//each hair-dot will be between .7 & .9 * the size of the previous one
h = h*random(.7,.9);
//the hair-dots will show up at random angles between 0 and 360 degrees
float a = random(0,360);
theta = radians(a);
// All recursive functions must have an exit condition!!!!
// Here, is the size of the circle, 15 pixels or less
if (h > 15) {
pushMatrix(); // Save the current state of transformation (i.e. where are we now)
rotate(theta); // Rotate by theta
ellipse(-h, -h, h, h); // Draw the circle
fill(0);
// translate(0, -h); // Move
hair(h); // Ok, now call myself to draw two new hair-dots
popMatrix(); // Whenever we get back here, we “pop” in order to restore the previous matrix state
// noFill();
// Repeat hair
pushMatrix();
rotate(-theta);
ellipse(h, h, -h, -h);
fill(0);
translate(0, -h);
hair(h);
popMatrix();
}
}